Gliding Bird
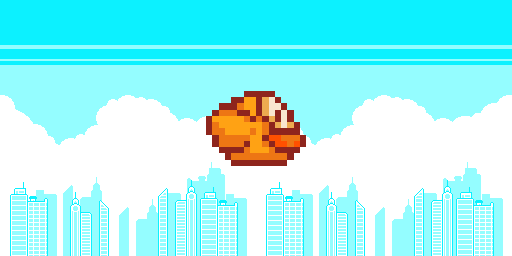
I like participating in Game Jams, and I like Linux, so when the Linux Game Jam 2022 came around, I wanted to participate. However, due to other commitments, I had a very limited amount of time to work on the project, so I knew I had to do something simple.
I decided to try and remake Flappy Bird, the old mobile game that the world became addicted to for a while. Unfortunately, things didn't go as planned...
One thing that I did to challenge myself was to not use an existing game engine, and instead build the game from scratch. As part of this, I had to implement collision, which I did using AABB
, or Axis-Aligned Bounding Box
. My implementation was simple, but it worked.
namespace FB
{
bool checkCollision(int x1, int y1, int h1, int w1, int x2, int y2, int w2, int h2)
{
if (x1 < x2 + w2 &&
x1 + w1 > x2 &&
y1 < y2 + h2 &&
y1 + h1 > y2)
return true;
return false;
}
bool checkPipeCollision(int birdX, int birdY, int pipeX, int pipeGap, int windowHeight)
{
return checkCollision(birdX, birdY, 16, 16, pipeX, 0, 32, pipeGap - 48) ||
checkCollision(birdX, birdY, 16, 16, pipeX, pipeGap + 48, 32, windowHeight);
}
} // namespace FB
One issue that I didn't really notice, was that I hadn't done the gravity calculations correctly, which has resulted in the bird not falling, but instead gliding. This is why I later changed the name to Gliding Bird, although most places still refer to the game's original name.
Overall, it was a fun experience, and it taught me how valuable time management can be during a jam. If you want to check out the game, it's linked down below.